Exploring Analog Outputs - ESP32
- automatelabonline
- Mar 1
- 3 min read
It is very common to find PWM outputs in microcontrollers. This type of output switches between high and low levels at a high frequency, so that the pin can act as an analog output in some cases, such as LEDs for example.
However, on the ESP32, there are two DAC (digital to analog converter) pins. These pins can generate truly analog outputs. These pins are GPIO25 (DAC1) and GPIO26 (DAC2).
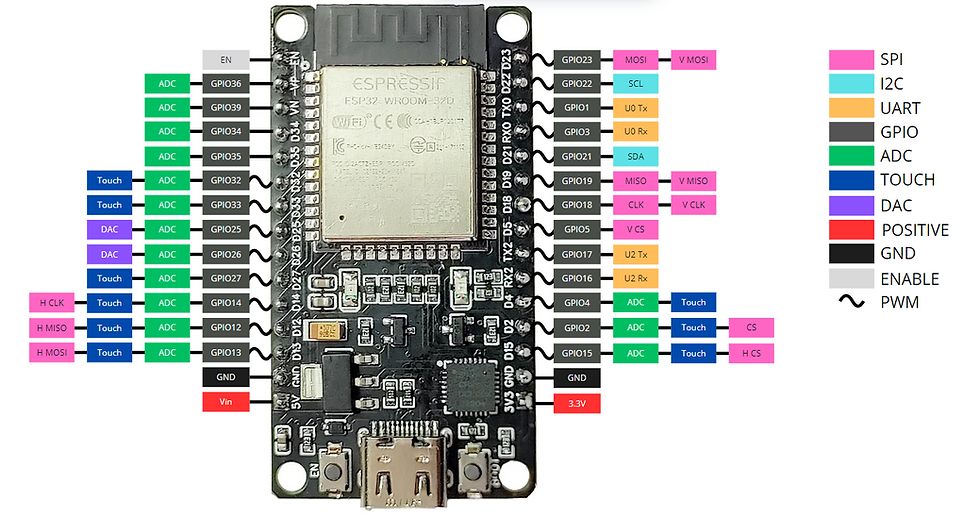
The ESP32 analog pins have 8-bit resolution, meaning they can generate 2⁸ = 256 different voltages, ranging from 0V to 3.3V. This means that the microcontroller can write voltages with a minimum range of:
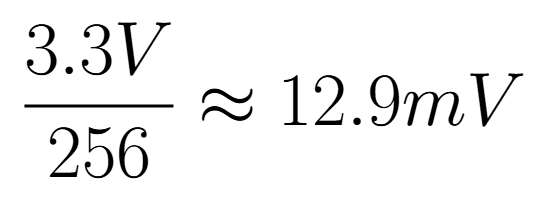
Functions for ESP32 Analog Outputs
The main function for using analog outputs is:
dacWrite(PIN, VALUE);
Where PIN is the GPIO for writing, which can be 25 or 26, and VALUE is an integer between 0 and 255 that represents the desired voltage for the pin. Given this desired voltage ( Vd ), the value passed to the function (let's call it D ) must follow the formula below.

For example, if the desired voltage at GPIO25 is 1V. Do:
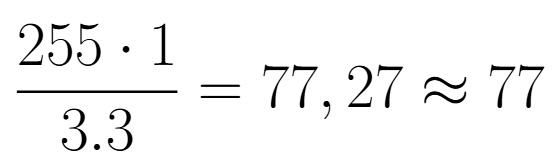
The result should always be rounded to the nearest integer. So the function to apply 1V to this pin is:
dacWrite(25, 77);
Project - Controlling the Brightness of an LED
We have already seen how to control the brightness of an LED using the PWM pins. However, with the DAC pins, it is possible to do this with a truly analog signal. To do this, we will use the circuit below. A potentiometer is read and controls the brightness of the LED. The LED is connected to pin 25 and the potentiometer to pin 4.
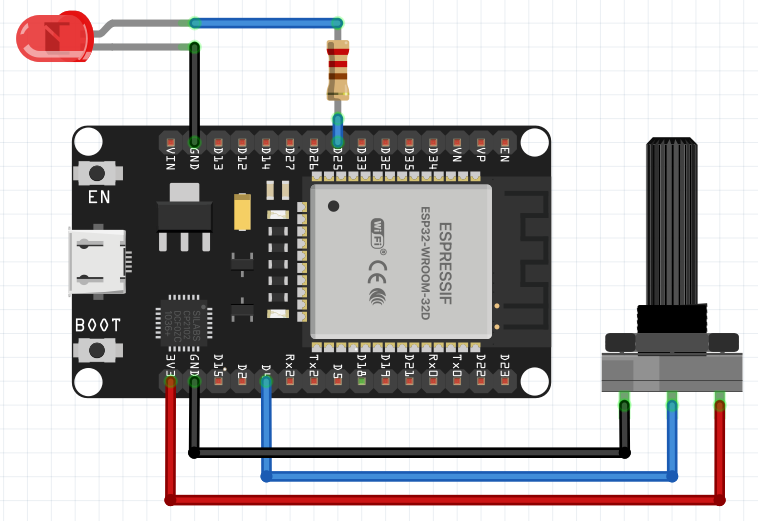
The code for the project is below.
#define PIN_LED 25 // define the LED pin
#define PIN_POTENTIOMETER 4 // define the potentiometer pin
void setup() {
}
void loop() {
int ledIntensity = map(analogRead(PIN_POTENTIOMETER), 0, 4095, 0, 255);
dacWrite(PIN_LED, ledIntensity); // Send the signal to the LED
delay(10);
}
Understanding the Code
First, the potentiometer read and LED write pins are defined.
#define PIN_LED 25 // define the LED pin
#define PIN_POTENTIOMETER 4 // define the potentiometer pin
In the loop() function, the potentiometer pin is read using the analogRead() function. This reading serves as input for another function, map(). This function passes the value from one range to another. In this case, the reading from the analog pin is 12 bits, so the returned value will be between 0 and 4095. However, the written value from the analog output is only 8 bits, so it goes between 0 and 255.
The map() function adjusts the value to this new range. For example, if the value read was 2047 (half between 0 and 4095), the function's output would be 127 (half between 0 and 255). The map() function follows the following format:
map(VALOR, IN_MIN, IN_MAX, OUT_MIN, OUT_MAX);
Where VALUE represents the input that will be transformed, IN_MIN and IN_MAX the input ranges and OUT_MIN and OUT_MAX the output ranges. Therefore, to map between the potentiometer reading ( IN ) and the analog output writing ( OUT ), the following is used:
int ledIntensity = map(analogRead(PIN_POTENTIOMETER), 0, 4095, 0, 255);
This value is passed as a parameter to the dacWrite() function, which sends the signal to the LED.
dacWrite(PIN_LED, ledIntensity); // Send the signal to the LED
Conclusions
In conclusion, the ESP32's analog outputs (DAC) offer an excellent option for generating analog signals accurately and efficiently, expanding the microcontroller's application possibilities in projects that require continuous signal control.
The ESP32’s ability to generate high-resolution signals and the flexibility of its DAC pins make it an attractive choice for systems that require audio, volume control, signal modulation, or analog interfaces. Its integration with other advanced features, such as Wi-Fi and Bluetooth, reinforces the ESP32’s potential for automation, audio, and Internet of Things (IoT) projects. By understanding and utilizing the ESP32’s DAC outputs, developers can create innovative solutions that combine performance, efficiency, and accessibility across a variety of technology areas.
Comments